The first Tkinter article showed how to create a simple window with a label. Here is the code:
import tkinter as tk
root = tk.Tk()
root.title("My first Tkinter window")
root.geometry("400x200")
root.grid_columnconfigure(0, weight=1)
tk.Label(
root,
text="Hello, World!",
background="green",
foreground="white",
font=("TkDefaultFont", 18),
).grid(row=0, column=0, padx=40, pady=40, sticky="nsew")
root.mainloop()
Grid was used as layout manager. After executing this code, this window appears:
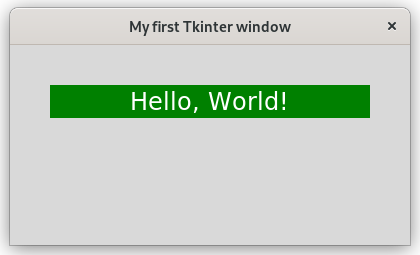
This simple app could now be further developed by adding more widgets. However, the more components are added, the more confusing the code becomes. Therefore, it may be useful to take an object-oriented approach. This blog post shows what this could look like.
For the main window and the label widget, a class called HelloWorldWindow
is used. The init
function of this class sets the title and window size:
def __init__(self, root):
"""Initialize the window
Args:
root (Tkinter object): Main window object
"""
self.root = root
root.title("My first Tkinter window")
root.geometry("400x200")
root.grid_columnconfigure(0, weight=1)
self.create_widgets()
The create_widgets
method is responsible for creating the corresponding label widget. In this example, grid is again used as layout manager:
def create_widgets(self):
"""Create Label widget for the window"""
self.label = tk.Label(
self.root,
text="Hello, World!",
background="green",
foreground="white",
font=("TkDefaultFont", 18),
)
self.label.grid(row=0, column=0, padx=40, pady=40, sticky="nsew")
Now all that’s missing is the creation of the window object and the execution of the event loop:
if __name__ == "__main__":
"""
Create the main window object and run the main event loop
"""
root = tk.Tk()
window = HelloWorldWindow(root)
root.mainloop()
And finally here is the complete code:
import tkinter as tk
class HelloWorldWindow:
def __init__(self, root):
"""Initialize the window
Args:
root (Tkinter object): Main window object
"""
self.root = root
root.title("My first Tkinter window")
root.geometry("400x200")
root.grid_columnconfigure(0, weight=1)
self.create_widgets()
def create_widgets(self):
"""Create Label widget for the window"""
self.label = tk.Label(
self.root,
text="Hello, World!",
background="green",
foreground="white",
font=("TkDefaultFont", 18),
)
self.label.grid(row=0, column=0, padx=40, pady=40, sticky="nsew")
if __name__ == "__main__":
"""
Create the main window object and run the main event loop
"""
root = tk.Tk()
window = HelloWorldWindow(root)
root.mainloop()