As a beginner in a programming language, you will hardly deal with the topic of code formatting. However, the more the project grows and 100 lines of code become 1000, you should think about formatting your code. The same applies when several programmers work on the same project. Each of them may use a different style of code and these different styles limits the readability significantly. This is the time when Black enters the stage. This code formatter is likely to be the most common. It’s easy to use and does a great job.
The installation is done with pip:
$ pip install black # Windows
$ pip3 install black # Linux, macOS
From now on, a single file can be formatted with the following command:
$ black <file name>
Now let’s take a look at a simple Python program that can be used to convert Arabic numerals to Roman numerals:
val = [1000,900,500,400, 100, 90, 50, 40, 10, 9, 5, 4, 1]
rom = ['M', "CM", "D", "CD", "C", "XC", "L", "XL", "X", "IX", "V", "IV", "I"]
def arabic_to_roman(arabic_number: int) -> str:
ans = ""
for i in range( 13 ):
while arabic_number >= val[i]:
print( i )
print(arabic_number, val[i])
ans += rom[i]
arabic_number-=val[i]
return ans
print(arabic_to_roman( 18 ))
If you save this code in a code editor such as Sublime Text or Visual Studio Code and, for example, use flake8 as Linter, the “errors” in the code will be shown to you:
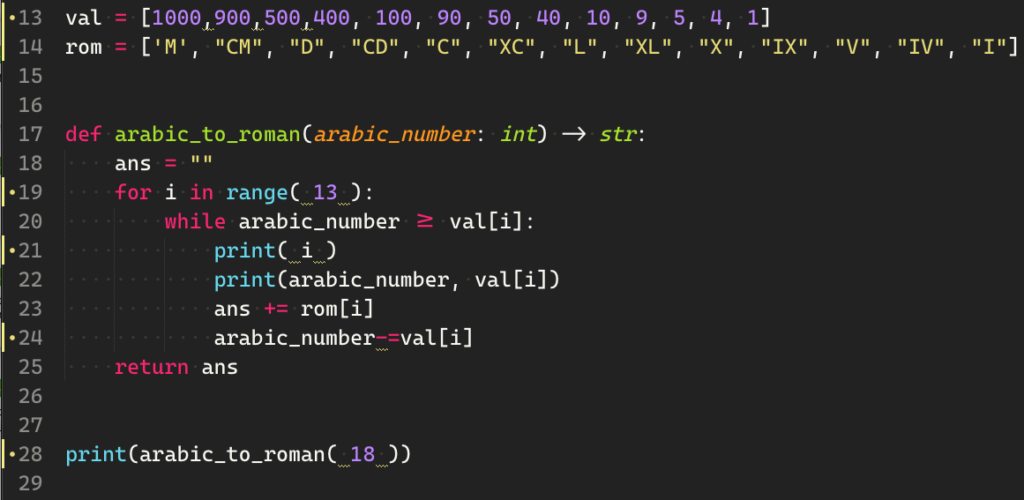
Issues are shown as wavy underlines in the editor. In addition, some yellow dots appear on the left. These formatting errors can be corrected manually. But why should this effort be made when a program is available for this? So, instead fixing every error step by step use black
:
$ black roman_numbers.py
reformatted roman_numbers.py
All done! ✨ 🍰 ✨
1 file reformatted.
Let’s see what happened:
val = [1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1]
rom = ["M", "CM", "D", "CD", "C", "XC", "L", "XL", "X", "IX", "V", "IV", "I"]
Black added a space after each element. The two lists now have a uniform appearance. Regarding the formatting used, black is based on the style guide defined in PEP8 (Python Enhancement Proposal 8), written in 2001 by Guido van Rossum. More information about the code style can be found on the PEP8 website.
In this example, black
not only added spaces, but also replaced the single quotes with double quotes. The linter didn’t complain, but black
prefers double quotes. The documentation for Black contains more information about the code style used.
If several files are to be formatted, the following syntax should be used:
$ black <directory>
This will reformat all files in the specified directory.
With the following command you can display what changes would be made to a file:
$ black --check --diff <Dateiname>
Now you know black, there is no reason not to make your code more readable.